How to Integrate “No CAPTCHA reCAPTCHA” in CodeIgniter Site
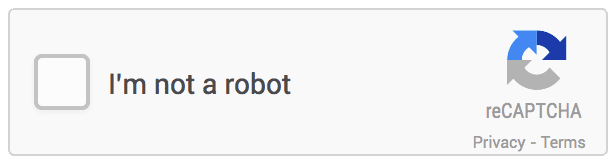
First, we need an API key, so head on over to https://www.google.com/recaptcha/admin. To gain access to this page you’ll need to be logged into a Google account. You’ll be asked to register your website, so give it a suitable name, then list domains (for example 1stcoder.com) where this particular reCAPTCHA will be used. Sub domains (such as dev.1stcoder.com and demo.1stcoder.com) are automatically taken into account.
After add the site, you’ll be given a site key and its partner secret key:
Now copy the site key and secret key to your CodeIgniter config.php file:
$config['use_recaptcha'] = TRUE; $config['recaptcha_sitekey'] = 'enter-your-site-key'; $config['recaptcha_secretkey'] = 'enter-your-secret-key';
Place the following script tag at the bottom of your page:
<script src='https://www.google.com/recaptcha/api.js'></script>
Next up is the placeholder which you’ll need to add to your form markup wherever you want the reCAPTCHA to appear:
<?php if($this->config->item('use_recaptcha')) { ?> <div class="form-group"> <label>Captcha:</label> <div class="g-recaptcha" data-size="normal" data-sitekey="<?php echo $this->config->item('recaptcha_sitekey'); ?> "></div> </div> <?php } ?>
Validate the Captcha on server side:
Download the Google_recaptcha.php file and upload it to your libraries folder(CURL needs to be enabled on the server)
Download Google reCaptcha Library
Add the following code to your controller, where you validate the form.
if($this->config->item('use_recaptcha')) { $this->form_validation->set_rules('g-recaptcha-response', 'Captcha', 'trim|xss_clean|required|callback__check_recaptcha'); }
And add the following validation callback function to your controller.
/** * Callback function. Check if reCAPTCHA test is passed. * * @return bool */ function _check_recaptcha($grecaptcha) { $this->load->library('google_recaptcha'); $gcaptcha = trim($grecaptcha); if(!empty($gcaptcha) && isset($gcaptcha)) { $siteKey = $this->config->item('recaptcha_sitekey'); $secret = $this->config->item('recaptcha_secretkey'); $lang = 'en'; $userIp=$this->input->ip_address(); $response = $this->google_recaptcha->getCurlData( $secret, $gcaptcha, $userIp ); $status= json_decode($response, true); if($status['success']){ return TRUE; } else { $this->form_validation->set_message('_check_recaptcha', $this->lang->line('recaptcha_validation_failed')); return FALSE; } } }
That’s all you have successfully added the google’s new “No CAPTCHA reCAPTCHA” to your CodeIgniter site with server side validation. You can now view the captcha on your form page in action.